Laravel Metadata
A PHP package for Laravel
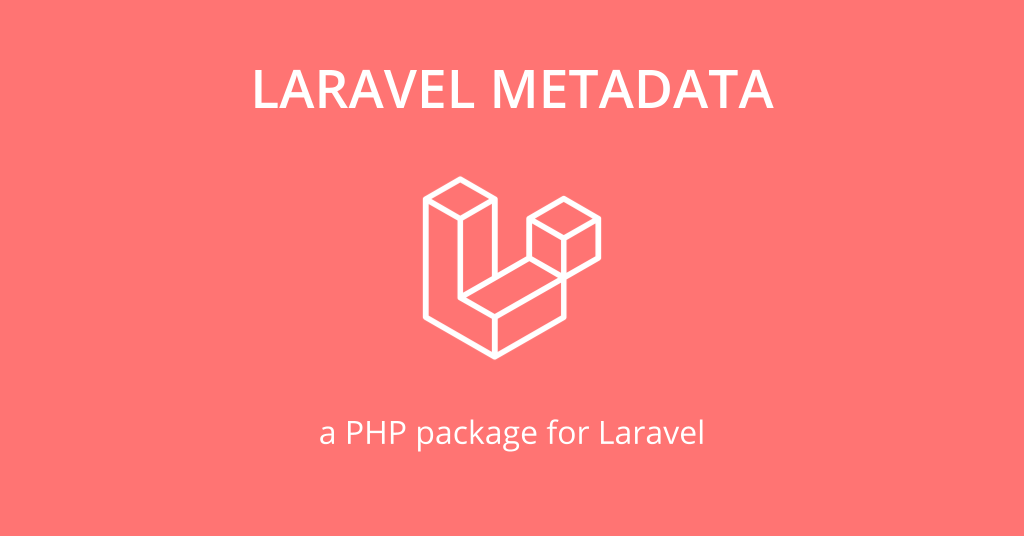
Laravel Metadata is a lightweight PHP package that helps you to handle extra data on your models without adding any new fields on your database table.
In many of my Laravel projects I had the need to store less relevant data and, instead of creating dozens of fields, I took inspiration from wordpress and how it handles metadata.
Thanks to the power of the Laravel polymorphism relations, the laravel-metadata package uses just one table for handling the metadata for all your models. What you have to do is just import a trait in your model.
If you take a look at the source code of the package you will see how simple and light is code.
Installation
Open the terminal in your laravel project and install the package below via composer:
composer require danielefavi/metadata
Then open the file config/app.php
and add the entry DanieleFavi\Metadata\MetaServiceProvider::class
in the providers
section:
'providers' => [
// ...
DanieleFavi\Metadata\MetaServiceProvider::class,
],
Then run the migration:
php artisan migrate
Import the metadata trait into your model
After installing the package you have to add the trait HasMetadata
in your model.
For example:
use DanieleFavi\Metadata\HasMetadata; // to add in your model
class User extends Authenticatable
{
use HasFactory;
use HasMetadata; // to add in your model
// ...
}
Now your model has all the methods to handle the metadata.
Examples
Saving the metadata
Using the method saveMeta
you can save (store or update) a metadata:
$user->saveMeta('phone_number', '111222333'); // storing a value
$user->saveMeta('color_preference', ['orange', 'yellow']); // storing an array
Getting the metadata
The method getMeta
retrieve the metadata for the given key:
$phoneNumber = $user->getMeta('phone_number');
// Default value in case the metadata has not been found (default: null)
$anotherMeta = $user->getMeta('another_meta', 10);
You can retrieve the metadata in bulk using the getMetas
method. The function getMetas
returns an array key-value containing the specified metadata key; for example:
$metas = $user->getMetas(['phone_number', 'address']);
The $metas
of the example above is going to have the following result:
[
'phone_number' => '111222333',
'address' => '29 Arlington Avenue'
]
If you instead want to get all the metadata just use $user->getMetas();
The list of methods goes on! You can find the list and examples on the github repository page _