Matrix arithmetic PHP Class
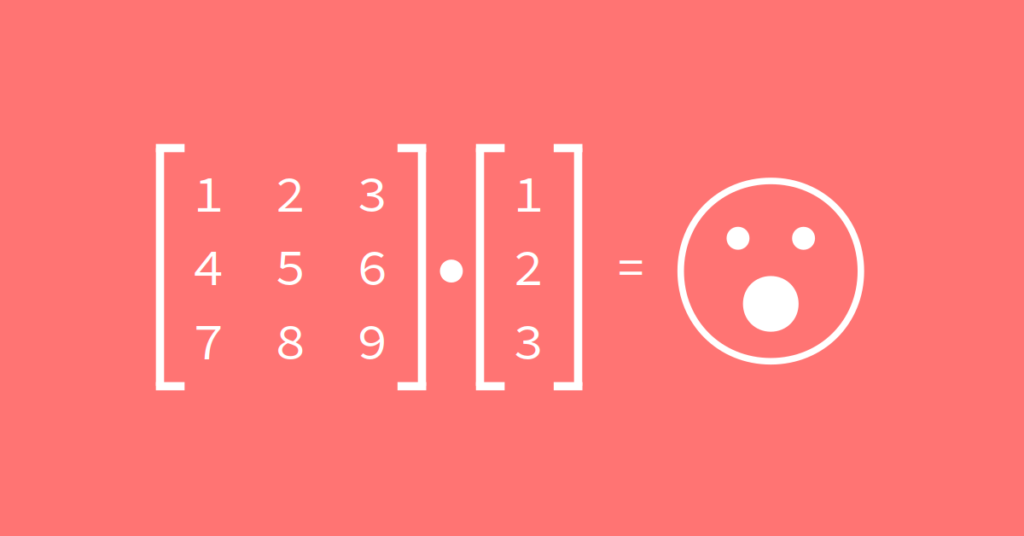
During my studies in AI I wanted to solve the XOR problem; I wanted just to do a quick test so I started writing the code in PHP. Solving that problem I ended up to write a class that handles matrix operations as well.
Since there is no reason to use AI with PHP (I’ll explain this in another article) I share with you the class that handle the matrix operations.
In this article I’m going to show you some of the methods of the class Matrix and how to use them.
With this class you can sum or subtract two matrices, dot products or product by value, transpose and many other operations.
In the following examples is assumed that the file Matrix.php is included and the Matrix
class is instantiated as $lib
:
$lib = new Matrix;
As Numpy (a Python library), the matrix is an array of arrays; a matrix is defined as following:
include “Matrix.php"
$lib = new Matrix;
// matrix 3×3
$matrix = [
[1 , 2 , 3],
[4 , 5 , 6],
[7 , 8 , 9]
];
// matrix 3×1 with a row
$matrix = [
[1 , 2 , 3]
];
// vector
$vector = [
[1],
[2],
[3]
];
// scalar
$scalar = [
[15]
];
// or more simply
$scalar = 15;
Matrix Transpose
The method matrixTranspose
returns the transpose of a matrix. Example:
$matrix = [
[1 , 2],
[3 , 4]
];
$matrix_transp = $lib->matrixTranspose($matrix);
sm($matrix_transp); // it shows the matrix the result in a nice way
Dot product
The function matrixDotProduct
has in input two matrices and it gives in output the dot product. If the two matrices are not compatible with each other an exception will be thrown.
$m1 = [
[1 , 2 , 3],
[4 , 5 , 6]
];
$m2 = [
[1 , 2],
[3 , 4],
[5 , 6]
];
$result = $lib->matrixDotProduct($m1,$m2);
sm($result); // it shows the matrix the result in a nice way
RELU, SIGMOID, TANH, LOG operation
The method matrixOperation
performs in each element of the given matrix the operation: RELU, SIGMOID, LOG (natural logarithm), NEGATIVE, TANH. In the following example we are going to perform SIGMOID to each element of $matrix
:
$matrix = [
[1 , 2],
[4 , 5]
];
$result = $lib->matrixOperation('sigmoid’, $matrix);
sm($result);
// This is what sm shows
// | 0.731 0.881 |
// | 0.982 0.993 |
Multiplie a matrix for a scalar value
The method matrixTimesValue
multiplies a scalar value to all the elements of a given matrix. The first parameter is the matrix and the second is the number (or a matrix 1×1 dimension).
$matrix = [
[1 , 2],
[4 , 5]
];
$result = $lib->matrixTimesValue($matrix, 10);
// Result of $matrix * 10
// | 10 20 |
// | 40 50 |
Matrix addition
The method matrixSum
performs the addiction of two gives matrices as parameters.
$m1 = [
[1 , 2],
[3 , 4]
];
$m2 = [
[11 , 12],
[13 , 14]
];
$result = $lib->matrixSum($m1, $m2);
// Result
// | 12 14 |
// | 16 18 |
Product value by value
This is a different from DOT product; it multiplies two matrices with same dimensions value by value _
$m1 = [
[1 , 2],
[3 , 4]
];
$m2 = [
[2 , 3],
[5 , 4]
];
$result = $lib->matrixProductValueByValue($m1, $m2);
// Result
// | 2 6 |
// | 15 16 |