WordPress Empty Plugin
How to create a Wordpress plugin
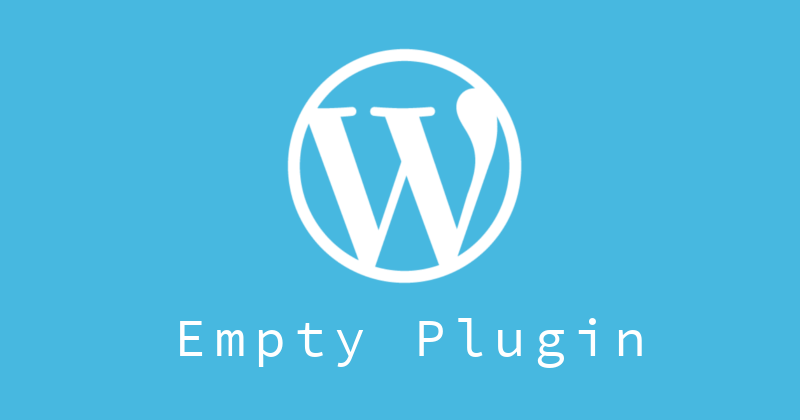
When I have to create a new WordPress functionality I always use an empty plugin to start with. Here I’m going to explain how to develop your WordPress plugin. If you are not interested in this tutorial you can just download the empty plugin:
STEP 1: create the plugin
First of all choose a name for your plugin: in this tutorial is Empty Plugin; let’s create a folder called empty-plugin inside wp-content/plugins. Then in this folder create a PHP file with the same name of the plugin folder; in my case I named it empty-plugin.php. To make WordPress recognize your plugin, you have to place the following comment in the head of the file you just created:
<?php
/*
Plugin Name: Empty plugin
Plugin URI: http://www.yourwebsitename.com/visit_plugin_website
Description: An empty wordpress plugin
Author: John Doe
Author URI: http://www.yourwebsitename.com/plugin_by
Version: 1.0.0
*/
If go to the plugin section you can see your plugin ready to be activated:

STEP 2: create the menu entries
The following code creates the entry Empty Plugin in the administration menu and the sub entry Add new:
function empty_plugin_create_menu_entry() {
add_menu_page('Empty Plugin’,
'Empty Plugin’,
'edit_posts’,
'main-page-empty-plugin’,
’empty_plugin_show_main_page’,
plugins_url('/images/empy-plugin-icon-16.png’, __FILE__) );
add_submenu_page('main-page-empty-plugin’,
'Add New’,
'Add New’,
'edit_posts’,
'add-edit-empty-plugin?action=add_new’,
’empty_plugin_add_another_page’ );
}
add_action('admin_menu’, ’empty_plugin_create_menu_entry’);
where add_menu_page
inserts the entry in the admin menu:
add_menu_page($page_title, $menu_title, $capability, $menu_slug, $function, $icon_image)
- Page title (required): in my case Empty Plugin
- Menu title (required): in my case Empty Plugin
- Capability (required): the capability of the logged user must have to interact with the plugin (for more info click here: https://codex.wordpress.org/Roles_and_Capabilities)
- Menu slug (main-page-empty-plugin): a unique slug to identify the menu
- Function that has to be triggered (my case: empty_plugin_show_main_page): function name to output the page content (check the STEP 3)
- Icon image path
To add a sub menu entry we used add_submenu_page
:
add_submenu_page($parent_slug, $page_title, $menu_title, $capability, $submenu_slug, $function)
- Parent menu slug: this field links the sub menu entry with the parent menu.
- Page title
- Menu title
- Capability
- Submenu slug
- Function to trigger to output the content for this page (check the STEP 3)
Now, we have to tell to wordpress to execute our function empty_plugin_create_menu_entry
when it is loading the admin menu:
add_action('admin_menu’, ’empty_plugin_create_menu_entry’);
with add_action
you are attaching the function named empty_plugin_create_menu_entry
to the action admin_menu
.
This is the final result:
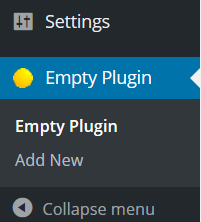
STEP 3: create the plugin pages
When we used add_menu_page
we choose to trigger the function empty_plugin_show_main_page
and in add_submenu_page
we choose to trigger empty_plugin_add_another_page
; so let’s declare these two functions:
function empty_plugin_show_main_page() {
include('main-page-empty-plugin.php’);
}
function empty_plugin_add_another_page() {
include('another-page-empty-plugin.php’);
}
The files included within the two functions above contains the PHP/HTML code of the page. In the administration pages you can also include CSS and JS files in this way:
wp_enqueue_style(’empty-plugin-style’, plugins_url( '/css/style.css’, __FILE__ ) );
wp_enqueue_script(’empty-plugin-scripts’, plugins_url( '/js/scripts.js’, __FILE__ ) );
If you write those two functions in the beginning of an administration page (eg: main-page-empty-plugin.php) you include the CSS and the JS file only in that administration page.
But if you write those two functions in the main plugin file you will include the CSS/JS file in all the pages of your website. So be very careful were you place wp_enqueue_style
and wp_enqueue_script
.
Other tips
To avoid to declare two different functions with the same name, you can add a prefix to your functions like my_plugin_get_option()
.
When you build your plugin don’t put PHP, CSS and JS file in the same folder; give a clean structure to the plugin grouping every kind of file in a different folder.
Don’t write all the classes and functions in one file only; better to give to each class its own file _